Saving Reconstruction(s) Under a Different Name#
If the existing output options from the nabu-slice or nabu-volume widgets are too limited, or if you want to copy a volume under another name (e.g., to reflect a filter used from Nabu reconstruction parameters), you can add a Python widget after the nabu-slice or nabu-volume reconstruction widget to modify the output name or copy the reconstructions.
Here is a small example. We consider a Python-script widget added just after the nabu-slice reconstruction widget:
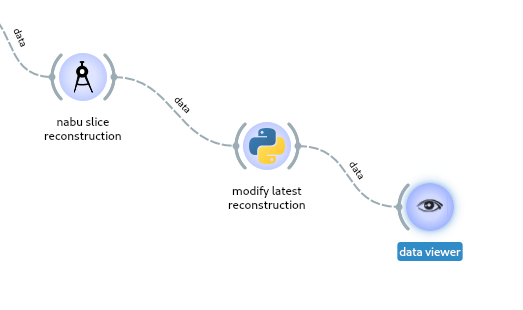
import os
from tomwer.core.volume.volumefactory import VolumeFactory
from tomwer.core.volume.hdf5volume import HDF5Volume
scan = in_data
new_reconstructions = []
# Go through all the latest 'nabu slice reconstruction' output
# Note: To go through all reconstructed volumes (after 'nabu-volume' widget)
# you can use scan.latest_vol_reconstructions instead. The rest is the same.
for identifier in scan.latest_reconstructions:
try:
# Retrieve the slice or the volume (same API)
in_volume = VolumeFactory.create_tomo_object_from_identifier(identifier)
in_volume.load()
except Exception:
print(f"Failed to load {in_volume}. Did the reconstruction fail?")
else:
# If you want, you can retrieve Nabu reconstruction parameters used.
nabu_config = scan.nabu_recons_params
# Copy the slice to another name (based on Nabu parameters)
# In this case, we want to know which FBP filter has been used.
filter_name = nabu_config["reconstruction"]["fbp_filter_type"]
# Then we create the new file name (in this case, we work with HDF5).
output_volume_dir = os.path.dirname(in_volume.file_path)
file_name = os.path.splitext( # Split file name and extension.
os.path.basename(in_volume.file_path) # Retrieve file name (without the path).
)[0]
new_file_path = os.path.join(
output_volume_dir,
f"{scan.scan_dir_name()}_{filter_name}.hdf5",
)
out_volume = HDF5Volume(
file_path=new_file_path,
data_path=in_volume.data_path,
data=in_volume.data,
metadata=in_volume.metadata,
)
print("New output volume file path is", new_file_path)
out_volume.save()
new_reconstructions.append(new_file_path)
# Register the new reconstructions (ensure downstream processing works from the new files).
if len(new_reconstructions) > 0:
scan.set_latest_reconstructions(
new_reconstructions
)
out_data = scan
Note
In this example, we go through all reconstructed slices using scan.latest_reconstructions. You can do the same for volumes. In this case:
The Python script widget must be placed after a ‘nabu-volume’ widget.
You must replace iteration over slices (scan.latest_reconstructions) with iteration over volumes (scan.latest_vol_reconstructions).
Set the latest reconstructed volume by calling set_latest_vol_reconstructions instead of set_latest_reconstructions.
Hint
If you are working with other volume types (e.g., TIFFVolume), please refer to the tomoscan volume API and the tomoscan volume tutorials. Tomwer uses the same API, but ensure you import the volume class from tomwer.core.volume instead of tomoscan.esrf.volume.