Remove reconstructed volumes#
Some users might want to delete reconstructed volume after some processing.
In this example we will take the use case that users want to: * to reconstruct the volume (using nabu volume) * cast the volume (ot 16bits) * delete the original (32 bits) volume
A widget exists for the first two tasks. We can create a python widget for the last one.
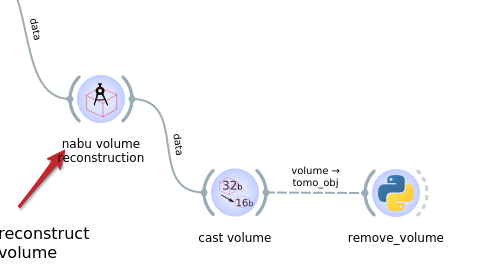
And we will link ‘cast volume’ data output to the python script in_data input.
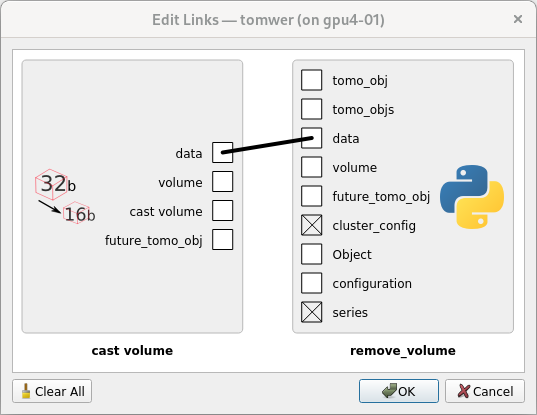
The following script will retrieve the ‘volume’ root path and simply remove it (according to it output format).
import shutil
import os
from tomwer.core.volume.volumefactory import VolumeFactory
from tomwer.core.volume.hdf5volume import HDF5Volume
scan = in_data
for volume_id in scan.latest_vol_reconstructions:
volume = VolumeFactory.create_tomo_object_from_identifier(volume_id)
if isinstance(volume, HDF5Volume):
# remove sub volumes
sub_file_dir, _ = os.path.splitext(volume.data_url.file_path())
shutil.rmtree(sub_file_dir, ignore_errors=True)
# remove master file
os.remove(volume.file_path)
else:
shutil.rmtree(volume.data_url.file_path(), ignore_errors=True)
Dealing with longer processing
As the python thread will wait for the deletion to be done before returning hand and as the python thread is executed in the main thread if you have large dataset to remove you might want to execute this in a thread.
import shutil
import threading
import os
from tomwer.core.volume.volumefactory import VolumeFactory
from tomwer.core.volume.hdf5volume import HDF5Volume
scan = in_data
def remove_volumes():
for volume_id in scan.latest_vol_reconstructions:
volume = VolumeFactory.create_tomo_object_from_identifier(volume_id)
if isinstance(volume, HDF5Volume):
# remove sub volumes
sub_file_dir, _ = os.path.splitext(volume.data_url.file_path())
shutil.rmtree(sub_file_dir, ignore_errors=True)
# remove master file
os.remove(volume.file_path)
else:
shutil.rmtree(volume.data_url.file_path(), ignore_errors=True)
thread = threading.Thread(target=remove_volumes)
thread.start()
remove_volume(scan.latest_vol_reconstructions)